For simple drawing, python provides a built-in module named turtle. This behaves like a drawing board and you can use various drawing commands to draw over it. The basic commands control the movement of the drawing pen itself. We start with a very basic drawing program and work our way through to draw a complete chess board using python turtle.
The following python program draws a square shape using the basic turtle commands,
import turtle
board = turtle.Turtle()
board.forward(100) # move forward
board.right(90) # turn pen right 90 degrees
board.forward(100)
board.right(90)
board.forward(100)
board.right(90)
board.forward(100)
board.right(90)
turtle.done()
This can be easily encapsulated into a simple box function as shown below,
import turtle
def draw_box(t):
for i in range(0,4):
board.forward(100) # move forward
board.right(90) # turn pen right 90 degrees
board = turtle.Turtle()
draw_box(board)
turtle.done()
But what if we want to draw different squares at different locations? The following python program shows how it can be done using turtle commands. The draw_box() function is enhanced to draw the square at a specific location. The following program draws a 3x3 grid of 30 pixel boxes.
import turtle
def draw_box(t,x,y,size):
t.penup() # no drawing!
t.goto(x,y) # move the pen to a different position
t.pendown() # resume drawing
for i in range(0,4):
board.forward(size) # move forward
board.right(90) # turn pen right 90 degrees
board = turtle.Turtle()
start_x = 50 # starting x position of the grid
start_y = 50 # starting y position of the grid
box_size = 30 # pixel size of each box in the grid
for i in range(0,3): # 3x3 grid
for j in range(0,3):
draw_box(board,start_x + j*box_size, start_y + i*box_size, box_size)
turtle.done()
Let us now enhance the above python program to draw a chess board. This program illustrates the use of color in turtle drawing,
import turtle
def draw_box(t,x,y,size,fill_color):
t.penup() # no drawing!
t.goto(x,y) # move the pen to a different position
t.pendown() # resume drawing
t.fillcolor(fill_color)
t.begin_fill() # Shape drawn after this will be filled with this color!
for i in range(0,4):
board.forward(size) # move forward
board.right(90) # turn pen right 90 degrees
t.end_fill() # Go ahead and fill the rectangle!
def draw_chess_board():
square_color = "black" # first chess board square is black
start_x = 0 # starting x position of the chess board
start_y = 0 # starting y position of the chess board
box_size = 30 # pixel size of each square in the chess board
for i in range(0,8): # 8x8 chess board
for j in range(0,8):
draw_box(board,start_x+j*box_size,start_y+i*box_size,box_size,square_color)
square_color = 'black' if square_color == 'white' else 'white' # toggle after a column
square_color = 'black' if square_color == 'white' else 'white' # toggle after a row!
board = turtle.Turtle()
draw_chess_board()
turtle.done()
Following is the chess board output from the above python program,
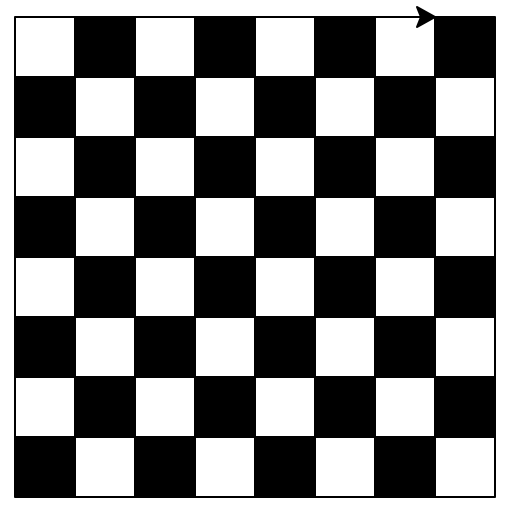